Video.js with React Hooks
A short guide to using Videojs with React Hooks.
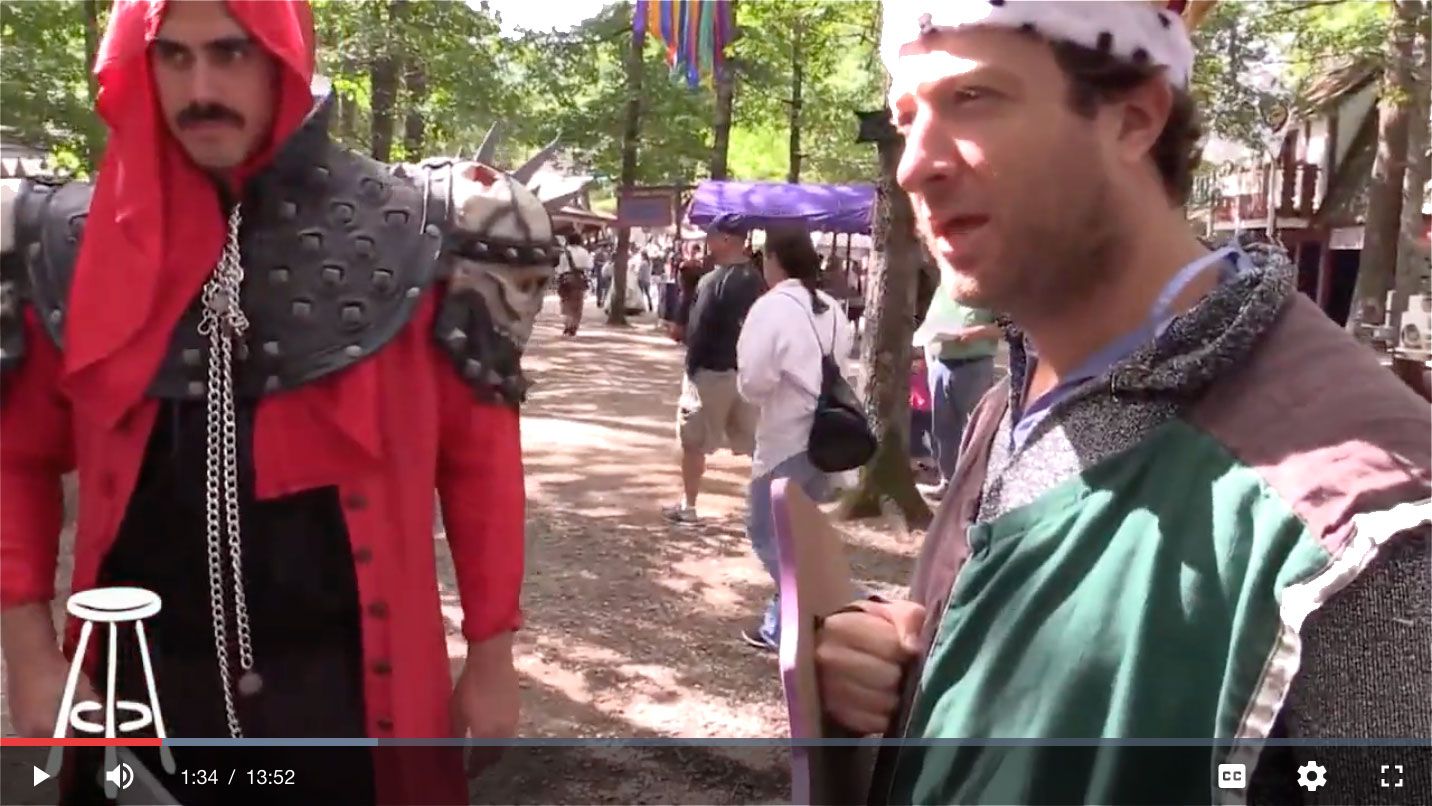
At Barstool Sports we use Video.js for video players across our web properties. We like the library for it's extensive feature set and customization capabilities but I've found that using Video.js with React can be a bit confusing. The Video.js documentation gives an example of how to use Video.js with React but the example uses class based components and is a bit dated so this article will guide you on how to use Video.js with React Hooks.
We'll start with a simple functional component called VideoPlayer
.
import React, { useEffect, useRef } from 'react'
import videojs from 'video.js'
import 'video.js/dist/video-js.css'
const VideoPlayer = ({ key, options }) => {
const container = useRef()
const player = useRef()
useEffect(() => {
player.current = videojs(container.current, options)
return () => {
player.current.dispose()
}
}, [key])
return (
<div data-vjs-player key={key}>
<video ref={container} />
</div>
)
}
export default VideoPlayer
The VideoPlayer
component initializes the Videojs player with the options passed as a prop that is properly disposed if the props change. Now you can Video.js methods with player.current
. For example, you could add an event listener for when the video plays with player.current.on('play', () => console.log('Video Play'))
.
Now we can use the VideoPlayer
like this:
import React from 'react'
const VideoPage = ({ video }) => {
const options = {
controls: true,
poster: video.poster,
sources: [{ src: video.url }]
}
return (
<VideoPlayer options={options} key={video.id} />
)
}
export default VideoPage
Setting a unique key
prop is important because our VideoPlayer
component will dispose and reinitialize when this is changed. Hopefully this helps with using Video.js with React Hooks in your project.